Learning Ethereum #1: Cracking the Calldata Code
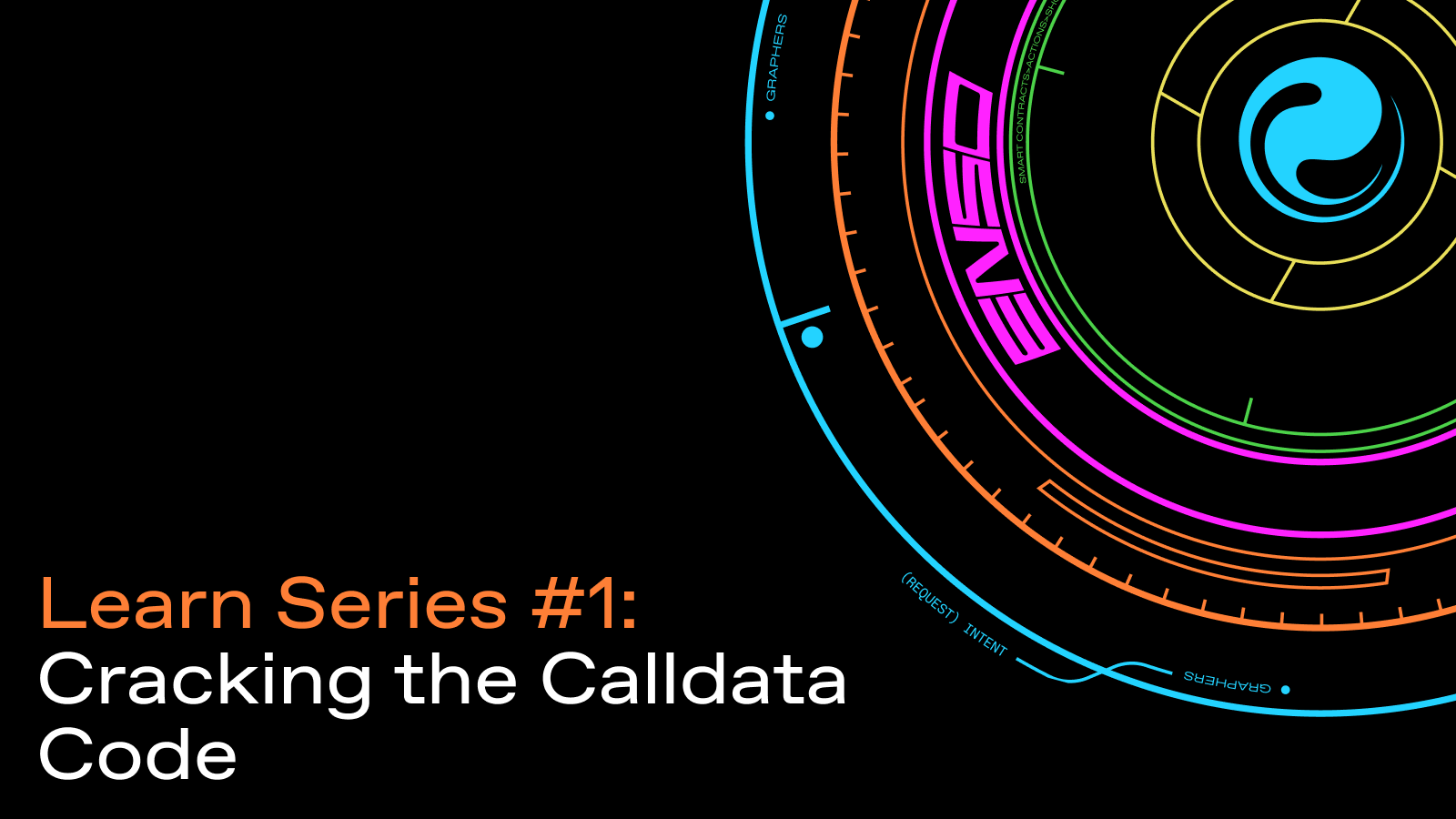
Ever seen those long, cryptic strings of characters in Ethereum transactions and wondered what they mean? Today, we're diving into the secret language of Ethereum: calldata.
What is Calldata?
Calldata is the encoded information sent to a smart contract when you interact with it. Think of it as instructions written in a special code that only the blockchain can understand. Every time you send tokens, swap on a DEX, or mint an NFT, you're sending calldata to a contract.

To understand why calldata exists, we need to know a bit about how Ethereum works:
- Smart Contracts: These are programs stored on the blockchain that run when predetermined conditions are met
- Transactions: To interact with smart contracts, users send transactions to the Ethereum network
- Gas Optimization: Data on Ethereum is expensive (it costs "gas"), so the data needs to be as compact as possible
- Universal Standard: Different programming languages need a common way to talk to the blockchain
Calldata solves these challenges by providing a standardized, compact format for encoding function calls and parameters. It's defined by something called the "Application Binary Interface" (ABI) - essentially a contract's communication protocol, but that topic is for another episode!
The Anatomy of Calldata
Let's break down a typical calldata string:
0x4910a1a500000000000000000000000000000000000000000000000000000000000000200000000000000000000000000000000000000000000000000000000000000009656e736f6275696c640000000000000000000000000000000000000000000000
When a calldata contains a string (like our challenge), it follows this encoding format:
- Function Selector: The first 8 characters after '0x'
- Data Offset: The next 64 characters tell us where the actual string data begins
- String Length: The next 64 characters tell us how long the string is
- The Actual String Data: The remaining part contains our message, encoded in hex
Function Selector Explained
The function selector is created by taking the first 4 bytes of the keccak256 hash of the function's signature. For example, if you're calling a function transfer(address,uint256), Ethereum hashes this text and takes the first 4 bytes as an identifier. This compact identifier tells the contract which function you want to execute.
What is a Data Offset?
The data offset is one of the most confusing parts of calldata. Ethereum needs a way to determine the location of this data when it has "dynamic" data types, such as strings or arrays, whose size isn't fixed. The offset is essentially a pointer indicating "jump ahead this many bytes to locate the actual data."In our example, the offset is 0x20 (which is 32 in decimal). This means "skip 32 bytes from the beginning of the parameters section to find the start of the string data."
Understanding Hexadecimal: 0x20 = 32
Let's break down how 0x20 equals 32:In hexadecimal (base-16), each digit position represents a power of 16:
- The rightmost digit represents 16⁰ (= 1)
- The next digit to the left represents 16¹ (= 16)
- The next represents 16² (= 256), and so on
For 0x20:
- The 0 digit is in the 16⁰ position, so: 0 × 1 = 0
- The 2 digit is in the 16¹ position, so: 2 × 16 = 32
Adding these up: 0 + 32 = 32 decimal. This is important because Ethereum processes data in 32-byte "words" - the fundamental storage unit in the Ethereum Virtual Machine (EVM). So the offset of 0x20 (32 bytes) means "skip exactly one EVM word."Think of it like chapters in a book; the offset is like saying, "Go to page 32 to start reading the chapter."
String Length Field
After jumping to the position specified by the offset, we find the string length field. This tells us exactly how many bytes our string takes up. In our example, it's 0x09 (9 in decimal), meaning our message is nine characters long. This is crucial because strings in Ethereum are padded to fill complete 32-byte slots, so we need to know exactly how many bytes are actual data versus padding. Let's walk through an example:
0xb80469eb (Function Selector for storeMessage(string))
0000000000000000000000000000000000000000000000000000000000000020 (Offset: 32 bytes)
0000000000000000000000000000000000000000000000000000000000000009 (Length: 9 bytes)
656e736f6275696c640000000000000000000000000000000000000000000000 (String Data)
To visualize this process:
- We start at the beginning of the parameters section (right after the function selector)
- The offset tells us to jump ahead 32 bytes
- At that position, we find the length (9 bytes)
- The next 9 bytes after that are our actual string data
- Any remaining bytes are just padding to fill the 32-byte slot
Hex to Text: The Final Step
The last part of our calldata contains the actual string data in hexadecimal format. But how exactly does hex represent text?
How Hex Encoding Works
Every character you see on your screen has a numeric code assigned to it by the ASCII or Unicode standard. For example:
- The letter 'a' is represented by the decimal number 97
- The letter 'b' is represented by 98
- Capital 'A' is 65
- And so on for every character
In computing, we often use hexadecimal (base-16) instead of decimal (base-10) to represent these numbers because it maps more efficiently to how computers store data. In hex:
- Decimal 97 ('a') becomes 61 in hex
- Decimal 98 ('b') becomes 62 in hex
- Decimal 65 ('A') becomes 41 in hex
Let's decode the example string:
- 65 → decimal 101 → 'e'
- 6e → decimal 110 → 'n'
- 73 → decimal 115 → 's'
- 6f → decimal 111 → 'o'
- 62 → decimal 98 → 'b'
- 75 → decimal 117 → 'u'
- 69 → decimal 105 → 'i'
- 6c → decimal 108 → 'l'
- 64 → decimal 100 → 'd'
When converted to ASCII text, the hex 656e736f6275696c64 becomes "ensobuild"!
Fun fact: This is exactly how ALL text is stored in computers, as numbers that map to characters according to standardized tables like ASCII.
Why This Matters
Understanding calldata isn't just for devs, it's useful for everyone in Web3.
Real-World Applications
- Security: Verify what you're actually doing before signing a transaction. Many scams involve tricking users into calling malicious functions. If you can decode calldata, you can see what you're actually approving.
- Debugging: When a transaction fails, the error might be in the calldata. Understanding how to read it helps you diagnose problems. For example, maybe you tried to send a token amount that exceeds your balance, and that parameter is visible in the calldata.
- Transparency: The blockchain is supposed to be transparent, but that's only true if people can read the data! Decoding calldata helps you verify that contracts do what they claim to do.
- Technical Understanding: Many Web3 concepts make more sense once you understand calldata. It's like learning the alphabet before trying to read books.
Try It Yourself! 🐈
Now it's your turn! Here's a challenge to try:
0xb80469eb0000000000000000000000000000000000000000000000000000000000000020000000000000000000000000000000000000000000000000000000000000000873686f7274636174000000000000000000000000000000000000000000000000
Can you decode the message? (Hint: It's an 8-character word)
The next time you see a transaction's input data, you'll know it's not just random characters. Blockchains are full of hidden messages if you know how to read them!
P.S. If you decoded our main challenge, congratulations! You're now officially a _________